Flutter hackathon #hack20 journey
I will share in this post how our Flutter hackathon #hack20 journey was. This is an anecdote on how the whole experience was for me and my team.
#hack20 background
#hack20 is the second of the annual Flutter hackathon hosted by the Flutter community that happened from 27-29th of June 2020. While it is not an official Google event, it is backed and sponsored by Google and other big companies in the field.
Basically, you think of a project that you can create using Flutter. You then form a team or work on your own for 48 hours before you submit your project. Top 20 winners get prizes, with the first place getting a $4000 worth of electronic setup.
The theme of your project can be Saving the Planet or Retro/Cyberpunk. There were around ~2600 participants, 650 teams and ~250 submitted projects
Did you win?
No, we did not 😂. There were two rounds of voting, and all participating teams get to vote on other teams’ projects. We luckily managed to reach the final round (one of the 90 teams), but that was it.
The project
You can checkout our code in this github repository. You can also try it on web from here, though some of the features are not really optimised for browsers. Make sure to use landscape! Also, exporting PDF on web sometimes work!
On top of that, here is the video that we almost did not submit due to iMovie crashing 30 minutes before the deadline.
The team
Our team of three is called GMT+10. We chose that name because we all work together here in Sydney, AU. The three of us are all Android developers who believed that we should use Flutter in our company app!
We believe that we are at a slight disadvantage because of timezones. The official time started at Saturday, 10:00 PM Sydney local time and will end at Monday 10:00 pm. So following the rules, we only have around 24 hours with sleep because we have a day job on a Monday.
Day 1 - Ideation
This is the fun part in my opinion. This is where we tried to think of ideas that can either be fun to use or just plain helpful to others. We used Invision to draw out and gather different ideas and suggestions, because all of us were working together remotely.
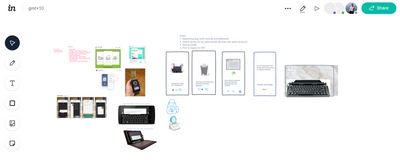
Our sketch before we started writing code
At the same time, this is the hardest part of the hackathon. There are so many considerations while we were thinking of possible projects.
- Is it doable in 48 hours?
- Will people use it?
- Has anyone already made one?
- Will someone possibly have the same project?
- Will it look presentable on the demo?
Each of these points are valid considerations. At the time, we did not know how voting will work, but I realized after that the video of the demo is the most important part of it.
On top of that, we avoided projects that other people will most likely make e.g. Recycling App, Retro Games, COVID tracing, etc.
P.S. The winner of the hackathon was actually a retro game (Game and Watch)
Day 2 - Making the app
This day is how we spent our time making the app. We were using Zoom to be constantly together. While everyone worked on every part of the code base, each one of us has a specific focus.
App design
As a disclaimer, I am in no means a designer. None of us in the team claimed to be. Therefore, our approach is to make the best design we can do with our skills. In order to do this, we have to go for the simplest thing we could do.
If you had a look at our ideation page above, the typewriter app would look something like this.
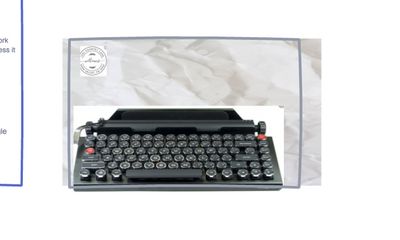
The keyboard is not even centered!
Because I have prior experience in using Sketch for images in my blog, I volunteered to make the designs and export them as assets.
One of the main source of assets that I used was unDraw. They are open source and don’t even require to cite them as sources. And if you haven’t already, don’t forget to give them a shoutout through twitter. I am sure that most of you have used one or two of their images.
I get these images, and modify them as I go. Even our app logo was derived from it. This is how my Sketch board looks like during the hackathon.
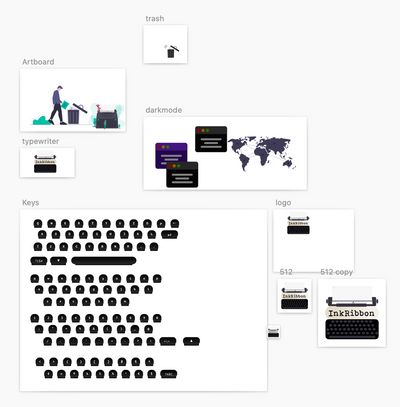
Messy messy sketchboard
Keyboard design
I designed the keyboard using Sketch. The idea is not an original one from me, as I have to look at how a typewriter keyboard would look in a mobile app. We used the top typewriter app in Play Store as a guide. Looking at our InVision screenshot again, here is what we used as a reference.
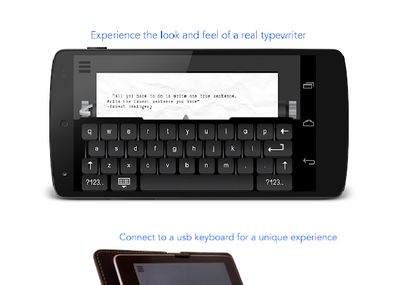
Existing typewriter app in Android
Now, the dreaded part is to design our own keyboard. I didn’t have much time and experience in doing this efficiently, so I had to quickly look for tutorials on how to do this properly.
In Sketch, there is a thing called Symbol, where it is like a master copy of a design. It means that if I change this master copy, all the designs that use this will also update. Here is what I had in Sketch.
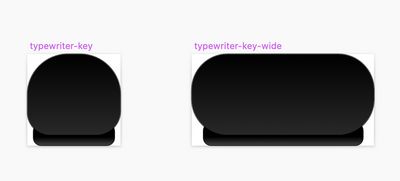
Far from perfect but I'm proud of it :)
Using that symbol, I had to make each key that will be present in the keyboard. The letters in the keyboard has to change their cases too, so I have to account for that as well. Never forget all those numbers and symbols!
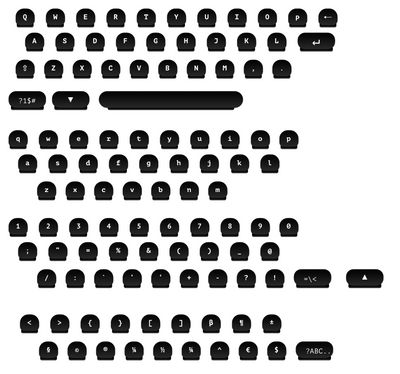
I already feel tired looking at it
Writing the code
For the most part, I wrote the code for the typewriter keyboard. It was far from perfect, and not really using any patterns such as BLoC.
What I did however is to make it reusable. The typewriter keyboard widget is just a normal Flutter widget that allows you to listen to keystroke events.
It means that user of this keyboard just needs to pass a TypewriterKeyboardController, listen to keys being pressed and process it elsewhere in the code. Here is an actual snippet of our main screen using the typewriter keyboard and controller.
void initState() {
super.initState();
_typewriterKeyboardController = TypewriterKeyboardController();
_typewriterKeyboardController.textStream.listen(_onTextReceived);
}
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
leading: BackButton(),
),
bottomSheet: TypewriterKeyboard(
typewriterKeyboardController: _typewriterKeyboardController,
),
);
}
void _onTextReceived(String text) {
final textValue = text.toLowerCase();
switch (textValue) {
//Update EditText
}
}
// © themobilecoder
Again, this could be further refactored, such that the logic to handle keys would be on a BLoC or a different service class. Given the time and pressure, we just wrote what would work at the time.
Code collaboration
This can a bit inconvenient sometimes. Each of us have our own style of writing code. While there is really no right or wrong with style, it is important to have a consistent way of structuring and formatting code within a codebase.
One example is line formatting. I personally prefer putting commas at the end of a Flutter tree code so it would look like a tree when auto format happens. Some of us however prefer to make it single line if it’s short enough.
Due to these inconsistencies, a small commit might end up having a large changeset due to spaces and formatting.
If we write code in Android, we will definitely have consistent style of writing code as we have been pair programming at work, and everyone just syncs with each other.
The good thing with what we did is that we barely stepped on each others’ toes. We split our work by features, such that one is working with Firebase authentication and the other is on exporting and viewing PDFs.
Day 3-ish - Submitting the project
The team was able to barely finish the app on Sunday evening. The problem is that the deadline will be at 10:00 pm Monday. We have our daily job on a weekday, so we only have the Monday afternoon to finish the video.
Making the video
We had an idea of just showing in the video how the app works, but it is so damn boring to present a note taking app with a typewriter design. I mean yeah, there’s Firebase integration so you can save your notes online, but every other note app does the same thing!
We already know that we are not going to win (not with that attitude). Our last saving grace is to just make a good video, and that’s what we tried to achieve.
Our solution is to select a really dramatic music that still sits well with the app, with a great message written in the note. Since we already knew we will never going to win, we decided to just write a message that wishes everyone good luck.
We were sure that other teams will be writing apps that will help save the planet, so that’s where we focused on. We spent a fair amount of time looking for a good music and thinking about a message.
If you have not watched the video above, here is the message in our video.
Our message to the hackathon participants
Exporting the video
Exporting the whole video gave us all a mild heart attack. It was 30 minutes before the deadline, and we just started exporting this movie project to a video file.
As someone who has not exported a 1-minute long video on a 6 year old laptop, this was a new experience for me. Our saving grace to our simple app is going down the drain as we saw this image. Oh, I could have benefited from the new Macbook prize 😂
We are in a dilemma because if I just force shutdown iMovie, it might take another 30 minutes to export. We are also not quite sure if we have saved the movie project, because iMovie does not have a manual Save function!!
Fortunately, the project was auto saved. One of my teammate was using a newer Macbook, so we thought of just copying the iMovie project (which was hard to locate btw), send it to him with our amazing Australian internet speed, and let him do the file export.
The miracle
While we are waiting for a miracle, my other team mate started to export the video in his laptop. I was browsing the Slack channel for this Flutter hackathon and saw a message that kept our hopes up.
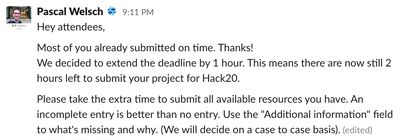
Just in time!
Now that we have an additional hour to submit our video, we knew that we will be able to sleep well that night.
Even before my teammates video export, iMovie successfully exported the video. However, iMovie didn’t recover so I just shut it down. If you have a dinosaur laptop like I do, stay away from iMovie.
Takeaway
It was a fun hackathon experience. I was coughing the whole time during the hackathon, so it was fun but not entirely a pleasant experience.
My teammates and I just proved that Flutter is a great tool to develop apps reliably on most platforms, including web. It will be a long stretch to convince our teammates in the office how much Flutter can do, but it does not matter.
I honestly learned a lot more in doing designs in Sketch. This is a skill that I really want to improve.
On the other hand, I am really impressed with the app ideas of the other participants, especially the ones with the Save the Environment theme.
Congratulations to everyone that won, and see you next hackathon!